Part 1: Setup
First, let's do some simple setup for the scaffolding for a NFT website.
Initial Setup
Create a directory and cd
into it:
mkdir nft-displayer && cd nft-displayer
Create an index.html
file:
touch index.html
Open up index.html in your favorite IDE / text editor and paste the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>NFT Displayer</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 20px;
}
#image-container {
margin-top: 20px;
}
</style>
</head>
<body>
<h1>NFT Displayer</h1>
<button onclick="fetchImage()">Load Image</button>
<div id="image-container"></div>
<script>
function fetchImage() {
// Placeholder API URL
const apiUrl = 'https://jsonplaceholder.typicode.com/photos/1';
const apiKey = ''
// Make a fetch request to the API
fetch(apiUrl)
.then(response => response.json())
.then(data => {
// Assuming the API returns an object with an 'url' property for the image
const imageUrl = data.url;
// Display the image in the #image-container div
document.getElementById('image-container').innerHTML = `
<img src="${imageUrl}" alt="API Image">
`;
})
.catch(error => {
console.error('Error fetching image:', error);
});
}
</script>
</body>
</html>
This code is a simple website with a button that (upon click) fetches an image from a placeholder URL.
View the Website
There are different ways to quickly spin up a server and view your website, includingVisual Studio Code with the Live Preview extension, which allows you to quick spin up a server and view your website. Initially, it'll look something like this:
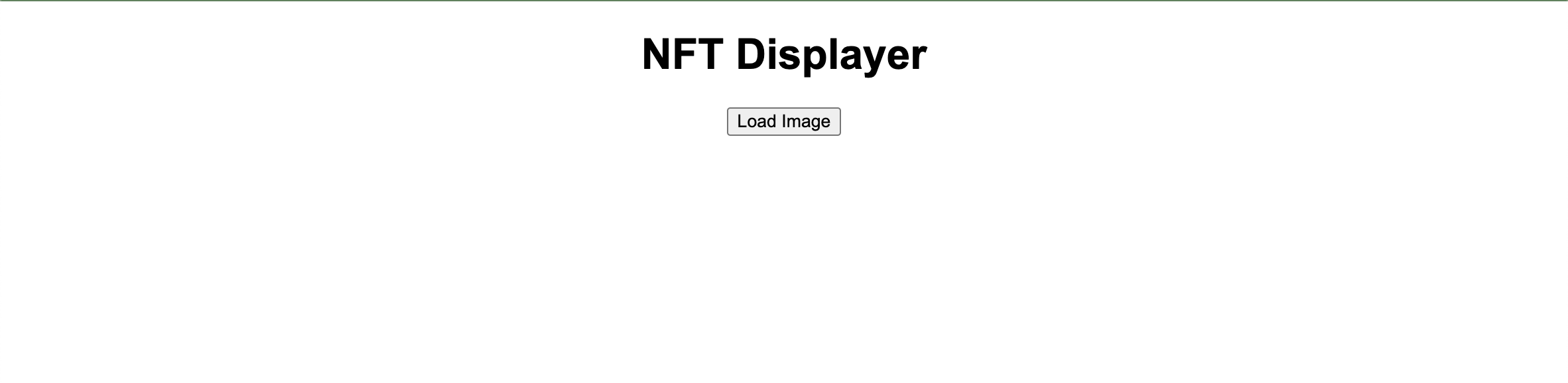
When you click "Load Image", it fetches a stock green image from an online service: json placeholder. That'll be fixed in subsequent steps.
Updated 3 months ago
What’s Next